lab 7 original
pdf
keyboard_arrow_up
School
Drexel University *
*We aren’t endorsed by this school
Course
171
Subject
Computer Science
Date
Feb 20, 2024
Type
Pages
13
Uploaded by ElderSquirrel3485
CS 171 - Lab 7
Professor Mark W. Boady and Professor Adelaida Medlock
Content by Professor Lisa Olivieri of Chestnut Hill College
Detailed instructions to the lab assignment are found in the following pages. •
Complete all the exercises and type your answers in the space provided. What to submit: •
Lab sheet in PDF. •
Screenshots with your code for questions 6, 5-k, 7 and 11 Submission must be done via Gradescope •
Please make sure you have tagged all questions to PDF pages and added your lab partner if any. •
We only accept submissions via Gradescope Student Name: Mustafa Bookwala Hitanshi Chhabria User ID (abc123): mmb479 hc866 Possible Points:
88
Your score out of 88
: Lab Grade on 100% scale:
Graded By (TA Signature):
CS 171 Lab 7 Week 7 2 Question 1: 3 points Create a text file named sports.txt
and enter the sports listed below, one word per line. Enter and execute the program below. Be sure the saved program is in the same folder as the text file. FYI:
In Python, you can access data from a text file as well as from the keyboard. You can create a text file in any text editing tool. You should only have one data item per line in the file. If you use Notepad (Windows) or TextEdit (Mac) make sure your settings are for Plain Text not Rich Text. You can also use Thonny to save a .txt
file Python Program 1 def
main() : 2 sportsList = open
(’sports.txt’)
3 sp = sportsList.
read
() 4 print
(sp) 5 6 ############ Call to main() ############ 7 main()
sports.txt basketball baseball football volleyball tennis golf soccer hockey swimming rowing
(a)
(1 point) What does the program do? The program calls the main() function that then firstly opens the ‘sports.txt’ text file
. The ‘.read()’ function then reads all of the text in the document as a single string and then prints it. (b)
(1 point) In the Line 2 of code, what does the string argument for the function open()
represent? The string argument for the function open() basically opens the ‘sports.txt’ text document
for the compiler. (c)
(1 point) Replace the call to the function read() with the function readline()
. Execute the program again. Explain the difference between the two functions: read()
and readline().
The read() function stores the entire text document as a string and the readline() function reads one line at a time. Both functions return a string, however.
CS 171 Lab 7 Week 7 3 Question 2: 10 points Enter and execute the following code. sportsList = open
(’sports.txt’)
for
index in range
(1, 11) : sp = sportsList.readline () print
( str
( index ) + ". ", sp)
(a)
(1 point) How does the output from this program differ from the output of the program that used the read()
function? In this program, we are basically printing each and every sport in a different line. The sport name is followed after the index and a dot. The output is like –
1. basketball 2. baseball 3. football 4. volleyball 5. tennis 6. golf 7. soccer 8. hockey 9. swimming 10. rowing (b)
(1 point) What caused the difference? The ‘.readline()’ function caused all the difference.
(c)
(1 point) What is the subtle difference in the output if the following print statement replaced the one above? print(index, ". ", sp)
The “print(index, ". ", sp)” causes an error when you run the program as index is an int type variable thus it cannot be concatenated with a string. (d)
(1 point) Which is better? For me, personally, the one which prints the index number is better as it makes it easy to read for me. (e)
(1 point) What does str(index)
do in the program above? The str(index) gives the output strings a numbering which makes it easy to read for the user.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
CS 171 Lab 7 Week 7 4 (f)
(1 point) Why is the str()
function necessary? As we are concatenating the variable index which is basically an integer, we need to first type cast it to a str to concatenate it without any errors. Hence, we use the str() function for that. (g)
(1 point) What happens when you change the arguments in the range()
function to 1, 10
? Upon changing the range() from 1 to 10, we do not get the last sport –
rowing –
printed when we run the updated code. (h)
(1 point) What happens when you change the arguments in the range()
function to 0, 30
? When the range() function is changed from 0 to 30, all the 10 sports are printed along with their index but after that only the index are printed from 11. to 29. The output after the last sport looks something like –
11. 12. . . . 29. (i)
(2 points) What do the results from (g) and (h) tell you about the arguments of the range()
function when you are reading data from a file with a for
loop? They tell me that the range() function runs from the starting index to the last –
1 index. For ex. If the range() function is from 1 to 21, only the values from 1 to 20 are executed. FYI:
The purpose of the rstrip()
function returns a copy of the string after all characters have been stripped from the end of the string (default whitespace characters, EOL characters, and newline characters). Question 3: 10 points The following program is slightly different from the program in Question 2. 1 sportsList = open
(’sports.txt’)
2 for
index in range
(1, 11) : 3 sp = sportsList.
readline ()
4 print
( str ( index ) + ". ", sp.rstrip () )
(a)
(2 points) Compare the output from this program to the previous program. What is the difference? Any extra spaces from the end of the string are stripped off from the text and are then printed. It makes the output look more professional and conventional. (b)
(2 points) What code caused the difference in the output? The ‘.rstrip()’ function c
aused the difference in the output.
CS 171 Lab 7 Week 7 5 (c)
(2 points) Does the rstrip()
function contain any arguments? No. The rstrip()does not contain any argument. (d)
(2 points) How does it know what string to act upon? It acts on the string that it is written before the dot. The dot operator tells it which text to strip spaces from. (e)
(2 points) lstrip()
is a similar function. What do you think it does? The lstrip() function strips space bars before the text written. Question 4: 6 points The following program is slightly different from the program in Question 3. 1 sportsList = open
(’sports.txt’)
2 for
index in range
(1, 11) : 3 sp = sportsList.
readline
() 4 if len
(sp) >= 8: 5 print
(sp.rstrip())
(a)
(2 points) How is the output different from Question 3 The above code prints the sport names of only those sports that are longer than 8 characters in length. (b)
(2 points) Two functions use what is known as dot (
.
) notation. What are the two functions? 1.
.readline() 2.
.rstrip() (c)
(2 points) Examine the output and explain what the len()
function does. The len() function calculates the length of every sport name from the sports.txt document. Question 5: 27 points Enter and execute the following program. 1 def main() : 2 lastName = input
("Enter last name: ") 3 firstName = input
("Enter first name: ") 4 studentID = input
("Enter ID: ") 5 6 inFile = open
("studentInfo.txt", "a") 7 inFile.
write
("Name: " + firstName + " " + lastName) 8 inFile.
write
("\nStudentID: " + studentID ) 9 inFile.
write
("\n") 10 inFile.
close
() 11 print
("Done! Data is saved in file: studentInfo.txt") 12 13 ############ Call to main() ############ 14 main()
CS 171 Lab 7 Week 7 6 (a)
(4 points) Which lines print to the screen? The lines that print to the screen are : line 11, that prints the ending statement Line 2, line 3 and line 4 also print to the screen although they are just input statements. (b)
(2 points) What does the program do? The program inputs the first name, last name, and student ID from the user. It then opens a ‘studentInfo.txt’ file and adds the Name and student ID to that file.
(c)
(2 points) Locate the file studentInfo.txt
on your computer. The file is stored in the same folder as the program. What is stored in the file? The data that is stored in the file studentInfo.txt says : Name: (Student’s first name) (Student’s Last Name)
Student ID: (Student’s student ID)
(d)
(2 points) Run the program again. How did the studentInfo.txt
file change? A new line was appended to the file with the new student’s information
(e)
(2 points) Change the argument "a" to "w" in the call to the open function. Run the program a third time. Explain what "a" to "w" do. On changing “a” to “w”, the student.txt file now contains only the most recent input’s information i.e. the argument “a” appends new lines to the text every time you run the program. The “w” argument just writes and replaces the previous data in the file on every run. (f)
(2 points) Did you need create the file studentInfo.txt
separately from the program code or did the program create it? The program created the file studentInfo.txt by itself. (g)
(2 points) Notice the function write()
. How many arguments does this function have? The function write(), contains just one string argument. (h)
(2 points) How does the write()
function know what file to write to?
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
CS 171 Lab 7 Week 7 7 The write() function is called by using the dot operator following the variable through which the desired file has been opened. For example, in the above code: inFile = open(
("studentInfo.txt", "a")
is written first and then the same variable is used to call the write() function. (i)
(2 points) What line of code closes the file? The “inFile.close()” in Line 10 closes the file.
(j)
(2 points) Where is the line of the code that closes the file positioned in the program? The line of code that closes the file is positioned at the end of the program after the main function has been defined and the data has been written to the file. (k)
(5 points) Write a program that prompts the user for information for three students: name, student ID, number of credits earned. Store the information in a file. You can use the above code as a template for your solution. Describe how you revised the code to solve this problem. Attach a screenshot showing your solution.
CS 171 Lab 7 Week 7 8 Application Questions Use the Python Interpreter to check your work.
Question 6: 5 points Write a program that randomly generates 1000 integers with values between 1 and 25. Writes the numbers to a file, one number per line. Seed the random number generator with the value 28. Put a screenshot of your source code and output text file. Only show part of your output file, you do not need to show all 1000 lines. You may submit more than one screenshot if needed to show all your source code.
CS 171 Lab 7 Week 7 9
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
CS 171 Lab 7 Week 7 10 Question 7: 5 points Write a program that reads the file you created in Question 6. Open the file and read in the values. Compute the Average, Minimum, and Maximum number from the file. Put a screenshot of your source code and output. You may submit more than one screenshot if needed to show all your source code.
CS 171 Lab 7 Week 7 11 Question 8: 4 points Examine the following program. It includes a function that takes a list as an argument. Enter and execute the code import
random def
orderList ( newList ) : newList.sort () newList.reverse () return
newList myList = [] for
y in range
(0, 100) : myList.append(random.randint (1, 100) ) print( orderList (myList) ) (a)
(2 points) What is the name of the function defined in this program? The name of the function defined in this program is orderList(newList): (b)
(2 points) What does the function do? The function sorts the list in ascending order and then returns the reversed list which is in descending order. Question 9: 4 points Enter and execute the following code.
CS 171 Lab 7 Week 7 12 userInput = input
("Enter a string that contains only letters: ") if
userInput.isalpha () : print
("Your string is valid.") else
: print
("Your string does not contain all letters.")
(a)
(2 points) Execute the program with different inputs and examine the output. Determine what the program does experimentally. Describe what this program does. This program asks the user to enter a string that takes only alphabets as an input. It does not even take in numbers in the string as input. (b)
(2 points) What does the isalpha()
function do? The isalpha() function checks if the string entered by the user has all alphabets or not. Question 10: 4 points Enter and execute the following code. numString = input
("Enter a number: ") if
numString.isdigit () : num = int
(numString) print
(num, "to the fourth power is", num ∗∗
4) else
: print
("Your input is not a valid number.") print
("Program terminated!") (a)
(2 points) Execute the program with at least five different types of input. Examine the output for each input. What does the program do? This program basically checks if the input given by the user is integer or not. If it is an integer, it typecasts the string input to an integer and then prints the fourth power of the number. If anything other than integers is entered as input, the program prints a statement saying that the user did not enter a valid number. My outputs were –
Input Output 2 2 to the fourth power is 16 name Your input is not a valid number. 23CS Your input is not a valid number. CS23 Your input is not a valid number. 4 4 to the fourth power is 256 (b)
(2 points) What does the isdigit()
function do? The isalpha() function checks if the string entered by the user has all numbers or not. Application Questions Use the Python Interpreter to check your work.
Question 11: 10 points
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
CS 171 Lab 7 Week 7 13 Download the following book from Project Gutenberg
: File 1404.txt from: http://www.gutenberg.org/files/1404/
Write a program that prints out every number in the file. Look at every line, then split into words. Print out any word where isdigit
returns True
. At the end of the program, print the total number of numbers found in the file. Submit screenshots of your code and its output. You may submit multiple screenshots if required to show all the source code and output.
Recommended textbooks for you
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
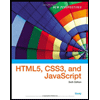
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Recommended textbooks for you
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
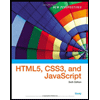
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning