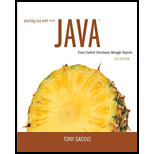
Starting Out with Java: From Control Structures through Objects (6th Edition)
6th Edition
ISBN: 9780133957051
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 7.7, Problem 7.16CP
Recall that we discussed a Rectangle class in Chapter 6. Write code that declares a Rectangle array with five elements. Instantiate each element with a Rectangle object. Use the Rectangle constructor to initialize each object with values for the length and width fields.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
how do you store multiple instances of a constructor in a javascript array and then print them out.
For example: I need to creat a class including the name, description and date and create 7 instances and store them in an array and them print them out.
This is the process of actually creating the array object and associating it
with the array variable. Give a one-word answer.
Task 1:The first task is to make the maze. A class is created called Maze, in which a 2D array for the maze is declared. Your job is to implement the constructor according to the given javaDoc. When you completed this task, write a set of Junit test case to test your code.As you probably noticed, the maze is defined as a private variable. The second job for you is to write an accessor(getter) method that returns the maze. Other information about what is expected for this method is given in the starter code.
public class PE1 {
MazedogMaze;
/**
* This method sets up the maze using the given input argument
* @param maze is a maze that is used to construct the dogMaze
*/
publicvoidsetup(String[][]maze){
/* insert your code here to create the dogMaze
* using the input argument.
*/
}
Chapter 7 Solutions
Starting Out with Java: From Control Structures through Objects (6th Edition)
Ch. 7.1 - Write statements that create the following arrays:...Ch. 7.1 - Whats wrong with the following array declarations?...Ch. 7.1 - Prob. 7.3CPCh. 7.1 - Prob. 7.4CPCh. 7.1 - Prob. 7.5CPCh. 7.1 - Prob. 7.6CPCh. 7.1 - Prob. 7.7CPCh. 7.1 - Prob. 7.8CPCh. 7.2 - Prob. 7.9CPCh. 7.2 - Prob. 7.10CP
Ch. 7.2 - A program has the following declaration: double[]...Ch. 7.2 - Look at the following statements: int[] a = { 1,...Ch. 7.3 - Prob. 7.13CPCh. 7.3 - Write a method named zero, which accepts an int...Ch. 7.6 - Prob. 7.15CPCh. 7.7 - Recall that we discussed a Rectangle class in...Ch. 7.10 - Prob. 7.17CPCh. 7.11 - What value in an array does the selection sort...Ch. 7.11 - How many times will the selection sort swap the...Ch. 7.11 - Prob. 7.20CPCh. 7.11 - Prob. 7.21CPCh. 7.11 - If a sequential search is performed on an array,...Ch. 7.13 - What import statement must you include in your...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Prob. 7.26CPCh. 7.13 - Prob. 7.27CPCh. 7.13 - Prob. 7.28CPCh. 7.13 - Prob. 7.29CPCh. 7.13 - Prob. 7.30CPCh. 7.13 - Prob. 7.31CPCh. 7 - In an array declaration, this indicates the number...Ch. 7 - Each element of an array is accessed by a number...Ch. 7 - The first subscript in an array is always. a. 1 b....Ch. 7 - The last subscript in an array is always. a. 100...Ch. 7 - Array bounds checking happens. a. when the program...Ch. 7 - This array field holds the number of elements that...Ch. 7 - Prob. 7MCCh. 7 - This search algorithm repeatedly divides the...Ch. 7 - Prob. 9MCCh. 7 - When initializing a two-dimensional array, you...Ch. 7 - Prob. 11MCCh. 7 - To delete an item from an ArrayList object, you...Ch. 7 - To determine the number of items stored in an...Ch. 7 - True or False: java does not allow a statement to...Ch. 7 - True or False: An arrays sitze declarator can be a...Ch. 7 - Prob. 16TFCh. 7 - True or False: The subscript of the last element...Ch. 7 - Prob. 18TFCh. 7 - True or False: The Java compiler does not display...Ch. 7 - Prob. 20TFCh. 7 - True or False: The first size declarator in the...Ch. 7 - Prob. 22TFCh. 7 - Prob. 23TFCh. 7 - int[] collection = new int[-20];Ch. 7 - Prob. 2FTECh. 7 - Prob. 3FTECh. 7 - Prob. 4FTECh. 7 - Prob. 5FTECh. 7 - The variable names references an integer array...Ch. 7 - The variables numberArray1 and numberArray2...Ch. 7 - Prob. 3AWCh. 7 - In a program you need to store the populations of...Ch. 7 - In a program you need to store the identification...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Prob. 9AWCh. 7 - Prob. 10AWCh. 7 - Prob. 11AWCh. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - Prob. 3SACh. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 7SACh. 7 - Prob. 8SACh. 7 - Prob. 9SACh. 7 - Rainfall Class Write a RainFall class that stores...Ch. 7 - Payroll Class Write a Payroll class that uses the...Ch. 7 - Charge Account Validation Create a class with a...Ch. 7 - Charge Account Modification Modify the charge...Ch. 7 - Prob. 5PCCh. 7 - Drivers License Exam The local Drivers License...Ch. 7 - Grade Book A teacher has five students who have...Ch. 7 - Grade Book Modification Modify the grade book...Ch. 7 - Prob. 10PCCh. 7 - Array Operations Write a program with an array...Ch. 7 - Name Search If you have downloaded this books...Ch. 7 - Population Data If you have downloaded this books...Ch. 7 - World Series Champions If you have downloaded this...Ch. 7 - 2D Array Operations Write a program that creates a...Ch. 7 - Prob. 17PCCh. 7 - Trivia Game In this programming challenge, you...Ch. 7 - Prob. 19PC
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
For each of the following activities, give a PEAS description of the task environment and characterize it in te...
Artificial Intelligence: A Modern Approach
Look at the following code, which is the first line of a class definition. What is the name of the superclass? ...
Starting Out with Python (3rd Edition)
Design a Do-Whi1e loop that asks the user to enter two numbers. The numbers should be added and the sum display...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Find out if your compiler supports variable-length arrays. If it does, write a small program to test the featur...
Programming in C
Explain the different aspects of the cost of a programming language.
Concepts Of Programming Languages
Code an SQL statement that creates a table with all columns from the parent and child tables in your answer to ...
Database Concepts (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Create a new class in the practicum1 package named ArrayUtilities. Write a method in the class that meets the following requirements: - It should be named "arrayToString" - It should declare a parameter for an int array. - It should return a String in the same format as the example above, e.g. "[1, 2, 3]". If the array is length 0, you should return the string "[]". - It should NOT use the Arrays.toString() method - you should create and return the string yourself. - You may receive a warning from IntelliJ about using string concatenation. You may ignore it. Write a main method to test your method by calling it with one or more small arrays and printing the string(s). Example output: [] [1, 2, 3] [2, 3, 5, 7, 11, 13]arrow_forwardWrite code for a test class with the following details:1. Create an object of each class mentioned in Question 3, assuming the values for the data members of each class. Create an array that takes the objects of ALL the classes as its elements. Write an enhanced for loop to display the object details. 2. the dealer in Question 4 decides to offer 2% percent discount on the commission at runtime. Write codewith explanation. 3. Add code to the test class that writes the invoice details of Question 6 to a txt document Syntax of the q3 code is given below: import java.util.*; class Car{ //class car String[] name; int reg_number,eng_number, chassis_num,status; String[] city, model, color, date_reg,date_arr,type; float price; float abstract calculate (float price); } class Dealer extends Car{ //inherits from Car String[] deal_name, deal_id, deal_add, deal_pno; float dealer_com ; float abstract calculate (float price) { dealer_com= 0.01*price +…arrow_forwardIN JAVA LANGUAGE Write an ATM class with an ArrayList of Account objects as an attribute. In the constructor, add 3 Account objects to your ArrayList. They can all have a start balance of $100 and an annual interest rate of 0.12. Include two methods, menu and makeSelection as outlined below. Please note that since both methods get user input, create a Scanner attribute to use in both. menu method This method does not have any parameters and does not return a value. It should: Get the account number from the user. This corresponds to the index of the items in the ArrayList. Since there are 3 elements in your ArrayList, you are going to ask them for a number between 1 and 3, but keep in mind that the indices of the ArrayList are 0-2, so you'll have to adjust the value you get from the user accordingly. Present the user with a main menu as shown below: Get their menu selection Call the makeSelection method, passing it the account index obtained in step 1 and the menu selection…arrow_forward
- Uising the code below. Write a test class called CircleTest that implement below specification: a). The class has an ArrayList that holds objects of type Circle. b). It displays a menu that allows the user to: Enter a Circle (the user only needs to enter the radius). Print all Circles (print the toString for each Circle in the ArrayList). Quit. c). If the user selects option 1, allow the user to input the radius of a circle. Place the circle in the ArrayList in order. You can do this by using loops and the CompareTo method. Do not use methods of ArrayList for this part. Use the compareTo method to insert elements into the array. For example addAt. d) To add a circle Cases: The ArrayList is empty The new circle is less than the first circle, add it at the beginning. The circle is greater than the last circle, add it at the end The new circle belongs somewhere in the middle. e). If the user selects option 2, loop through the ArrayList and print the toString for each member of the…arrow_forwardJava programming help please. Main and other class of code is below. Thank you so so much. i have to Write a program that does the following: You have an array of integers with positive and negative values. using one ourArray object (will post code of "ourArrary" below) , and in two loops maximum, How can you reorganize the content of the array so that all negative numbers are on one side and the positive numbers are on the other side. [-3, 2, -4, 5, -6, -8,-9, 7, 13] The output should be [-3, -4, -6, -8, -9, 2, 5, 7, 13] Our array code: public class ourArray<T> implements iQueue{ private T[] Ar; private int size = 0; private int increment = 100; //default constructor @SuppressWarnings("unchecked") public ourArray () { Ar = (T[]) new Object[100]; size = 0; } //constructor that accepts the size @SuppressWarnings("unchecked") public ourArray (int n) { Ar = (T[]) new Object[n]; size = 0; } //constructor that specifies the initial capacity and the increment…arrow_forwardWrite code that creates an ArrayList that can hold String objects. Add the names of three cars to the ArrayList, and then display the contents of the ArrayList.arrow_forward
- Challenge exercise Create a class called SortingTest. In it, create a method that accepts an array of int values as a parameter and prints the elements sorted out to the terminal (smallest element first).arrow_forward. Download the program from the instructor’s GitHub account (Ch3HW) then update it tocreate a class Student that includes four properties: an id (type Integer), a name (type String), a Major (type String) and a Grade (type Double). Use class Student to create objects (using buttonAdd) that will be read from the TextFields then save it intoan ArrayList. Perform the following queries on the ArrayList of Student objects and show the results on the listViewStudents (Hint: add buttons as needed):a) Use lambdas and streams to sort the Student objects by name, then show the results.b) Use lambdas and streams to map each Student to its name and grade, sort the results by grade (descending), then display the results.c) Use lambdas and streams to map each Student to its name and grade, to select the Students who have grade values in the range 80 to 90. Sort the results by grade value(descending), then show the results.d) Use lambdas and streams to calculate the total average of all Students…arrow_forwardYou are required to design an item class which has following attributes String name; Int stock; Int price; String expiry_Date; Provide default & Parameterized constructors. Provide getters & setters for data members. Provide a toString() method to print values. Now create an array of items store values in it (take values form user). After that you have to print the following All the items in the array. items whose name starts with ‘a’; All sold out items (whose stock is zero). All the items having expire date before 2-11-2020. Solve this using Simple java, arrays in java and string methods if required.arrow_forward
- Create a Golf Game. Allow for two players. Prompt for the player names. Each player plays 18 holes. Use an array or List for each player to hold their number of swings for each hole. Logical methods should be implemented for different tasks (broken out below). It is up to you to determine the parameters and return types of each method as appropriate. Method 1 - For each hole, the player has a number of swings. Determine the player's swings by generating a random number between 1 and 4. Store each players number of swings in the array/list. Method 2 - After completing the 18 holes, add up the number of swings for each hole for each player. The player with the fewest number of swings is the winner. Method 3 - Save the game data into a CSV file in the main project (root) directory. Name the file "Golf_Game_Player1_Player2.csv" using the player's names. The information in the file should look like this: playername, scoreforhole1, scoreforhole2, scoreforhole3, etc... Method 4 - In the…arrow_forwardPls, explain how this program question applies to the code below and comment on it in the program. for example, comment the which part of the question applies to the code and how it worksarrow_forwardWrite a code using Console.WriteLine for the image.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
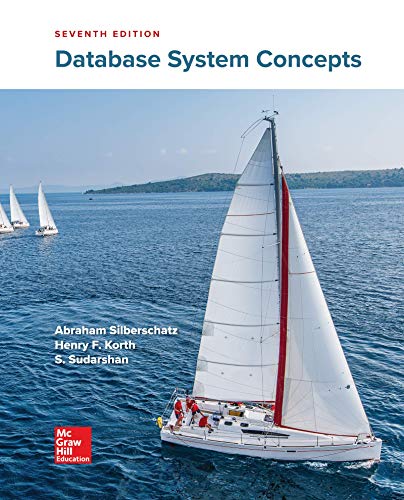
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
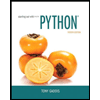
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
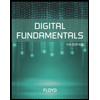
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
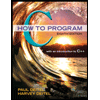
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
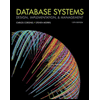
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
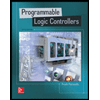
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Java Math Library; Author: Alex Lee;https://www.youtube.com/watch?v=ufegX5o8uc4;License: Standard YouTube License, CC-BY