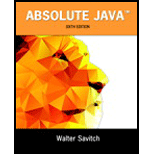
Concept explainers
Design and code a Swing GUI calculator. You can use Display 17.19 as a starting point, but your calculator will be more sophisticated. Your calculator will have two text fields that the user cannot change: One labeled "Result" will contain the result of performing the operation, and the other labeled "Operand" will be for the user to enter a number to be added, subtracted, and so forth from the result. The user enters the number for the "Operand" text field by clicking buttons labeled with the digits o through 9 and a decimal point, just as in a real calculator. Allow the operations of addition, subtraction, multiplication, and division. Use a GridLayout manager to produce a button pad that looks similar to the keyboard on a real calculator.
When the user clicks a button for an operation, the following occurs: the operation is performed, the "Result" text field is updated, and the "Operand" text field is cleared. Include a button labeled "Reset" that resets the "Result" to 0.0. Also include a button labeled "Clear" that resets the "Operand" text field so it is blank.
number in the "Operand" text field. Because values of type double are, in effect, approximate values, it makes no sense to test for equality with 0.0. Consider an operand to be "equal to zero" if it is in the range

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Absolute Java (6th Edition)
Additional Engineering Textbook Solutions
Mechanics of Materials (10th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Starting Out With Visual Basic (8th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- Please solve and answer the questions correctly please. Thank you!!arrow_forwardConsidering the TM example of binary sum ( see attached)do the step-by-step of execution for the binary numbers 1101 and 11. Feel free to use the Formal Language Editor Tool to execute it; Write it down the current state of the tape (including the head position) and indicate the current state of the TM at each step.arrow_forwardI need help on inculding additonal code where I can can do the opposite code of MatLab, where the function of t that I enter becomes the result of F(t), in other words, turning the time-domain f(t) into the frequency-domain function F(s):arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
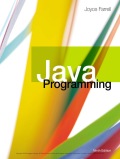
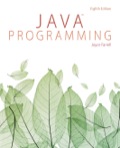
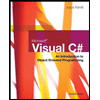
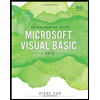