CS 410 Binary to C++ Activity Template
docx
keyboard_arrow_up
School
Southern New Hampshire University *
*We aren’t endorsed by this school
Course
410
Subject
Computer Science
Date
Feb 20, 2024
Type
docx
Pages
29
Uploaded by colossaltitan
CS 410 Binary to C++ Activity Template
File One
Step 2:
Explain the functionality of the blocks of assembly code.
Blocks of Assembly Code
Explanation of Functionality
0:
push %rbp
1:
mov %rsp,%rbp
4:
sub $0x10,%rsp
8:
movl $0x1,-0x8(%rbp)
f:
cmpl $0x9,-0x8(%rbp)
13:
jg a3 <main+0xa3>
19:
movl $0x1,-0xc(%rbp)
20:
cmpl $0x9,-0xc(%rbp)
24:
jg 9a <main+0x9a>
Allocates 16 bytes to the stack
Moves a value of 1 (32 bit / 4 bytes) to -0x8(%rbp)
Compares a value of 9 to -0x8(%rbp)
Jumps to a3
if the value at -0x8(%rbp) is greater than 9
Moves a 4 byte value of 1 to -0xc(%rbp)
Compares a value of 9 to -0xc(%rbp)
Jumps to 9a
if the value at -0x8(%rbp) is greater than 9 (after 9a the value at -0x8(%rbp) is incremented by one then there is a jump to f
)
26:
mov -0x8(%rbp),%eax
29:
imul -0xc(%rbp),%eax
2d:
mov %eax,-0x4(%rbp)
30:
mov -0x8(%rbp),%eax
33:
mov %eax,%esi
35:
lea 0x0(%rip),%rdi # 3c <main+0x3c>
3c:
callq 41 <main+0x41>
41:
lea 0x0(%rip),%rsi # 48 <main+0x48>
48:
mov %rax,%rdi
4b:
callq 50 <main+0x50>
Moves the value at -0x8(%rbp) to the 32 bit eax register
Multiplies the value at %eax by the value at -0xc(%rbp)
Moves the value at %eax to -0x4(%rbp)
Moves the value at -0x8(%rbp) to %eax
Moves %eax to %esi
Loads the effective address of the instruction pointer (0x3c) to %rdi
Calls a function at 0x41 with %rdi and %esi as the 1
st
and 2
nd
arguments
Loads the effective address of the instruction pointer (0x48) to %rsi
Moves %rax containing the value of the previous function call to %rdi
Calls a function at 0x50 with %rdi and %rsi as the 1st and 2nd arguments
50:
mov %rax,%rdx
53:
mov -0xc(%rbp),%eax
56:
mov %eax,%esi
58:
mov %rdx,%rdi
5b:
callq 60 <main+0x60>
60:
lea 0x0(%rip),%rsi # 67 <main+0x67>
67:
mov %rax,%rdi
6a:
callq 6f <main+0x6f>
6f:
mov %rax,%rdx
72:
mov -0x4(%rbp),%eax
Moves %rax containing the value of the previous function call to the 64 bit rdx
Moves the value at -0xc(%rbp) to the 32 bit eax register
Moves the value at %eax to %esi
Moves the value at %rdx (containing the value of the previous function call) to %rdi
Calls a function at 0x60 with %rdi and %esi as the 1st and 2nd arguments
Loads the effective address of the instruction pointer (0x67) to %rsi
Blocks of Assembly Code
Explanation of Functionality
75:
mov %eax,%esi
77:
mov %rdx,%rdi
7a:
callq 7f <main+0x7f>
Moves %rax containing the value of the previous function call to the 64 bit rdi
Calls a function at 0x6f with %rdi and %rsi as the 1st and 2nd arguments
Moves %rax containing the value of the previous function call to the 64 bit rdx
Moves the value at -0x4(%rbp) to the 32 bit eax register
Moves the value at %eax to %esi
Moves the value at %rdx (containing the value of the previous function call) to %rdi
Calls a function at 0x7f with %rdi and %esi as the 1st and 2nd arguments
7f:
mov %rax,%rdx
82:
mov 0x0(%rip),%rax # 89 <main+0x89>
89:
mov %rax,%rsi
8c:
mov %rdx,%rdi
8f:
callq 94 <main+0x94>
94:
addl $0x1,-0xc(%rbp)
98:
jmp 20 <main+0x20>
Moves %rax containing the value of the previous function call to the 64 bit rdx
Moves the value of the instruction pointer (0x89) to the 64 bit rax register
Moves the value at %rax to %rsi
Moves the value at %rdx (containing the value of the previous function call) to %rdi
Calls a function at 0x94 with %rdi and %rsi as the 1st and 2nd arguments
Increments the value at -0xc(%rbp) by 1
Jumps to 20
9a:
addl $0x1,-0x8(%rbp)
9e:
jmpq f <main+0xf>
a3:
mov $0x0,%eax
a8:
leaveq
a9:
retq
If the conditional jump at 24 is met (the value at -0xc(%rbp) is greater than 9) then 1 is added to -0x8(%rbp)
Jump to f
If the conditional jump at 13 is met (the value at -0x8(%rbp) is greater than 9) then a value of zero will be moved to %eax
Deallocates the stack frame with leave instruction and returns from the stack
Step 4: Convert the assembly code to C++ code.
Step 5: Explain how the C++ code performs the same tasks as the blocks of assembly code.
Blocks of Assembly Code
C++ Code
Explanation of Functionality
0:
push %rbp
1:
mov %rsp,%rbp
4:
sub $0x10,%rsp
8:
movl $0x1,-0x8(%rbp);
f:
cmpl $0x9,-0x8(%rbp)
13:
jg a3 <main+0xa3>
19:
movl $0x1,-0xc(%rbp)
20:
cmpl $0x9,-0xc(%rbp)
int main() { // enters main stack
int x4;
for (int x8 = 1; x8 <= 9; x8++) {
// … } return 0;
for (int xc = 1; xc <= 9; xc++)
{
// … } x8++;
First three instructions prepare the stack
The stack variable -0x8(%rbp) has a 32 bit move operation of 1 (32 bit=4bytes) int is usually 4 bytes (-0x8(%rbp) is represented as int x8).
Blocks of Assembly Code
C++ Code
Explanation of Functionality
24:
jg 9a <main+0x9a>
In a for loop because at the end of the loop (
9a
), the x8 variable is incremented by 1 and there is
jump back to
f. This jump is followed by a conditional jump to a3 (outside the main loop) if x8 is greater than 9 guaranteeing that x8 loops as long as it is not greater than 9.
The stack variable -0xc(%rbp) has a 32 bit move operation of 1 (32 bit=4bytes) int is usually 4 bytes (-0xc(%rbp) is represented as int xc).
For similar reasons, xc is in a for
loop however it increments (
94
)
then jumps to 20
to compare if xc is greater than 9. If it is then it jumps to 9a
which is then followed by an increment to the
x8 variable (end of first loop)
26:
mov -0x8(%rbp),%eax
29:
imul -0xc(%rbp),%eax
2d:
mov %eax,-0x4(%rbp)
30:
mov -0x8(%rbp),%eax
33:
mov %eax,%esi
35:
lea 0x0(%rip),%rdi # 3c <main+0x3c>
3c:
callq 41 <main+0x41>
41:
lea 0x0(%rip),%rsi # 48 <main+0x48>
48:
mov %rax,%rdi
4b:
callq 50 <main+0x50>
int x4 = X8 * xc;
std::cout << x8 << “ * “
Moves value at x8 to the eax register
the value at the eax register is multiplied by the value of xc
The multiplied value is moved to x4 (-0x4(%rbp) is represented as int x4)
Moves value at x8 to eax
Moves value at eax to esi
*Some pointers are stream objects such as std::cout or std::endl and most function calls resolve to std::basic_ostream<char, std::char_traits<char>>::operat
or<<() which can take in a string
stream as its 1
st
input. so the results of a previous function call are chained to subsequent
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
C++ Code
Explanation of Functionality
function calls f(f(f(p, int), int), int), int)
Loads the effective address of a the current address of the instruction pointer (location of some pointer in this case to std::cout) to the 64 bit rdi register
Calls a function located at 41 (<<(int)) with %rdi and %esi as the 1
st
and 2
nd
arguments.
Loads the effective address of a the current address of the instruction pointer (location of some pointer in this case to null
terminated string data “ * “) to the 64 bit rsi register.
Moves the value at %rax (the value of the previous function call) to %rdi
Calls a function located at 0x50 (<<) with %rdi and %rsi as the 1
st
and 2
nd
arguments
50:
mov %rax,%rdx
53:
mov -0xc(%rbp),%eax
56:
mov %eax,%esi
58:
mov %rdx,%rdi
5b:
callq 60 <main+0x60>
60:
lea 0x0(%rip),%rsi # 67 <main+0x67>
67:
mov %rax,%rdi
6a:
callq 6f <main+0x6f>
6f:
mov %rax,%rdx
72:
mov -0x4(%rbp),%eax
75:
mov %eax,%esi
77:
mov %rdx,%rdi
7a:
callq 7f <main+0x7f>
<< xc << “ = “ << x4;
Moves the value at %rax (the value of the previous function call) to %rdx
Moves the value of xc to %eax
Moves the value of %eax to %esi
Moves the value at %rdx to %rdi
Calls a function located at 0x60 (<<) with %rdi and %esi as the 1
st
and 2
nd
arguments
Loads the effective address of a the current address of the instruction pointer location of some pointer in this case to null
Blocks of Assembly Code
C++ Code
Explanation of Functionality
terminated string data “ = “) to the 64 bit rsi register.
Moves the value at %rax (the value of the previous function call) to %rdi
Calls a function located at 0x6f (<<) with %rdi and %rsi as the 1
st
and 2
nd
arguments
Moves the value at %rax (the value of the previous function call) to %rdx
Moves the value of x4 to %eax
Moves the value at %eax to %esi
Calls a function located at 0x7f (<<) with %rdi and %rsi as the 1
st
and 2
nd
arguments
7f:
mov %rax,%rdx
82:
mov 0x0(%rip),%rax # 89 <main+0x89>
89:
mov %rax,%rsi
8c:
mov %rdx,%rdi
8f:
callq 94 <main+0x94>
94:
addl $0x1,-0xc(%rbp)
98:
jmp 20 <main+0x20>
<< std::endl;
} // xc++ (inner for loop ends)
Moves the value at %rax (the value of the previous function call) to %rdx
Moves the value of the instruction pointer (std::endl) to
%rax
Moves %rax to %rsi
Moves %rdx to %rdi
Calls a function located at 94 () with %rdi and %rsi as the 1
st
and
2
nd
arguments.
Increments xc by 1 and jumps back to 0x20 indicating the end of the inner for loop
9a:
addl $0x1,-0x8(%rbp)
9e:
jmpq f <main+0xf>
a3:
mov $0x0,%eax
a8:
leaveq
a9:
retq
} // x8++ (outer for loop ends)
return 0;
} // end of int main()
Increments x8 by 1 and jumps back to 0x0f indicating the end of the outer for loop
Moves 0 to eax in preparation
Blocks of Assembly Code
C++ Code
Explanation of Functionality
for int main() to return 0
Deallocates the stack frame with leave instruction and
returns from the stack
File Two
Step 2:
Explain the functionality of the blocks of assembly code.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
Explanation of Functionality
0:
push %rbp
1:
mov %rsp,%rbp
4:
sub $0x30,%rsp
8:
mov %fs:0x28,%rax
f:
00 00
11:
mov %rax,-0x8(%rbp)
15:
xor %eax,%eax
The first three instructions allocate 48 bytes to the stack
Moves data at %fs:0x28 to %rax
Moves 64 bit value at %rax to -0x8(%rbp)
Xors %eax with itself effectively setting those bits to zero
17:
lea 0x0(%rip),%rsi # 1e <main+0x1e>
1e:
lea 0x0(%rip),%rdi # 25 <main+0x25>
25:
callq 2a <main+0x2a>
2a:
mov %rax,%rdx
2d:
mov 0x0(%rip),%rax # 34 <main+0x34>
34:
mov %rax,%rsi
37:
mov %rdx,%rdi
3a:
callq 3f <main+0x3f>
3f:
lea -0x14(%rbp),%rax
43:
mov %rax,%rsi
46:
lea 0x0(%rip),%rdi # 4d <main+0x4d>
4d:
callq 52 <main+0x52>
Loads the effective address of the instruction pointer to %rsi
Loads the effective address of the instruction pointer to %rdi
Calls a function located at 2a which takes %rdi and %rsi as the 1
st
and 2
nd
arguments
Moves the value at %rax (the value of the previous function call) to %rdx
Moves the value of the instruction pointer to %rsi
Moves the value at %rdx to %rdi
Calls a function located at 3f which takes %rdi and %rsi as the 1
st
and 2
nd
arguments
Loads the effective address of -0x14(%rbp) to %rax
Moves %rax to %rsi
Loads the effective address of the instruction pointer to %rdi
Calls a function located at 52 which takes %rdi and %rsi as the 1
st
and 2
nd
arguments
52:
mov -0x14(%rbp),%edx
55:
mov -0x14(%rbp),%eax
58:
imul %eax,%edx
5b:
mov -0x14(%rbp),%eax
5e:
imul %edx,%eax
61:
mov %eax,-0x14(%rbp)
64:
mov -0x14(%rbp),%eax
67:
cvtsi2sd %eax,%xmm0
6b:
movsd 0x0(%rip),%xmm1 # 73 <main+0x73>
72:
00
73:
mulsd %xmm1,%xmm0
77:
movsd %xmm0,-0x10(%rbp)
Moves the value at -0x14(%rbp) to the 32 bit edx register
Moves the value at -0x14(%rbp) to %eax
Multiplies the value at %edx by the value at %eax
Moves the value at -0x14(%rbp) to %eax
Multiplies the value at %eax by the value at %edx
Moves the value at %eax to -0x14(%rbp)
Moves the value at -0x14(%rbp) to %eax
Converts the signed integer at %eax to a scalar double and stores the converted floating point value at %xmm0
Blocks of Assembly Code
Explanation of Functionality
Moves the current value of the instruction pointer to %xmm1
Multiplies the value stored at %xmm0 by %xmm1
Moves the value at %xmm0 to -0x10(%rbp)
7c:
lea 0x0(%rip),%rsi # 83 <main+0x83>
83:
lea 0x0(%rip),%rdi # 8a <main+0x8a>
8a:
callq 8f <main+0x8f>
8f:
mov %rax,%rdx
92:
mov -0x10(%rbp),%rax
96:
mov %rax,-0x28(%rbp)
9a:
movsd -0x28(%rbp),%xmm0
9f:
mov %rdx,%rdi
a2:
callq a7 <main+0xa7>
Loads the effective address of the instruction pointer and stores it in% rsi
Loads the effective address of the instruction pointer and stores it in% rdi
Calls a function located at 8f that takes %rdi and %rsi as the 1
st
and 2
nd
arguments
Moves the value at %rax (the value of the previous function call) to %rdx
Moves the value at -0x10(%rbp) to %rax
Moves the value at %rax to -0x28(%rbp)
Moves the value at -0x28(%rbp) to %xmm0
Moves %rdx to %rdi
Calls a function located at a7 that takes %rdi as the 1
st
argument
a7:
mov $0x0,%eax
ac:
mov -0x8(%rbp),%rcx
b0:
xor %fs:0x28,%rcx
b7:
00 00
b9:
je c0 <main+0xc0>
bb:
callq c0 <main+0xc0>
c0:
leaveq
c1:
retq
Moves zero to eax
Moves the value at -0x8(%rbp) (which held %fs:0x28 at the start of the stack frame) to %rcx
Xors %fs:0x28 with the value at %rcx
If they are the same (which it should) deallocate the stack frame and return the function otherwise call a function c0 before the deallocation.
Step 4: Convert the assembly code to C++ code.
Step 5: Explain how the C++ code performs the same tasks as the blocks of assembly code.
Blocks of Assembly Code
C++ Code
Explanation of Functionality
0:
push %rbp
1:
mov %rsp,%rbp
4:
sub $0x30,%rsp
8:
mov %fs:0x28,%rax
f:
00 00
11:
mov %rax,-0x8(%rbp)
#include <iostream>
int main()
{
Allocates 48 bytes to the main stack frame
Blocks of Assembly Code
C++ Code
Explanation of Functionality
15:
xor %eax,%eax
17:
lea 0x0(%rip),%rsi # 1e <main+0x1e>
1e:
lea 0x0(%rip),%rdi # 25 <main+0x25>
25:
callq 2a <main+0x2a>
2a:
mov %rax,%rdx
2d:
mov 0x0(%rip),%rax # 34 <main+0x34>
34:
mov %rax,%rsi
37:
mov %rdx,%rdi
3a:
callq 3f <main+0x3f>
3f:
lea -0x14(%rbp),%rax
43:
mov %rax,%rsi
46:
lea 0x0(%rip),%rdi # 4d <main+0x4d>
4d:
callq 52 <main+0x52>
int x14;
std::cout << “Enter Radius:” << std::endl;
std::cin >> x14;
Loads the effective address of the instruction pointer (location
of some pointer in this case to null terminated string data “ Enter Radius:“) to the 64 bit rsi register.
Loads the effective address of the instruction pointer (location
of some pointer in this case to “std::cout“) to the 64 bit rdi register.
Calls a function located at 2a (<<) with %rdi and %rsi as the 1
st
and 2
nd
arguments
Moves %rax (the result of the function call) to the %rdx register
Moves the value of the instruction pointer (location of some pointer in this case to “std::endl“) to the 64 bit rax register
Moves the value at %rax to %rsi
Calls a function located at 3f (<<) with %rdi and %rsi as the 1
st
and 2
nd
arguments
Loads the effective address of -0x14(%rbp) (int x14)
to %rax
Moves %rax to %rsi
Loads the effective address of the instruction pointer (location
of some pointer in this case to “std::cin“) to the 64 bit rdi register.
Calls a function located at 52 (>>) with %rdi and %rsi as the 1
st
and 2
nd
arguments
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
C++ Code
Explanation of Functionality
52:
mov -0x14(%rbp),
%edx
55:
mov -0x14(%rbp),
%eax
58:
imul %eax,%edx
5b:
mov -0x14(%rbp),
%eax
5e:
imul %edx,%eax
61:
mov %eax,
-0x14(%rbp)
64:
mov -0x14(%rbp),
%eax
67:
cvtsi2sd %eax,%xmm0
6b:
movsd 0x0(%rip),
%xmm1 # 73 <main+0x73>
72:
00
73:
mulsd %xmm1,%xmm0
77:
movsd %xmm0,
-0x10(%rbp)
x14 = x14 * x14 * x14;
double x10 = ((double) x14) * 3.14
Moves the value of x14 to %edx
Moves the value of x14 to %eax
Multiplies the value at %edx by the value at %eax
Moves the value of x14 to %eax
Multiplies the value at %eax by the value at %edx
Moves the value at %eax to x14
Moves the value at x14 to %eax
Converts the signed integer value at x14 to signed double and moves it to %xmm0
Moves the value of instruction pointer (location of some pointer in this case to a constant double value of 3.14) to %xmm1
Multiplies the values at %xmm0
by %xmm1
Moves the value at %xmm0 to -0x10(%rbp) (double x10)
7c:
lea 0x0(%rip),%rsi # 83 <main+0x83>
83:
lea 0x0(%rip),%rdi # 8a <main+0x8a>
8a:
callq 8f <main+0x8f>
8f:
mov %rax,%rdx
92:
mov -0x10(%rbp),
%rax
96:
mov %rax,
-0x28(%rbp)
9a:
movsd -0x28(%rbp),
%xmm0
9f:
mov %rdx,%rdi
a2:
callq a7 <main+0xa7>
//…
std::cout << “The volume is: “;
double x28 = x10;
std::cout << x28;
Loads the effective address of the instruction pointer (location
of some pointer in this case to null terminated string data “The
volume is: “) to the 64 bit rsi register.
Loads the effective address of the instruction pointer (location
of some pointer in this case to std::cout) to the 64 bit rdi register.
Calls a function located at 8f (<<) with %rdi and %rsi as the
Blocks of Assembly Code
C++ Code
Explanation of Functionality
1
st
and 2
nd
arguments
Moves %rax (the result of the previous function call) to %rdx
Moves the value at x10 to %rax
Moves the value at %rax to
-0x28(%rbp) (double x28)
Moves the value at x28 to %xmm0
Moves the value at %rdx (the value of the previous function call) to %rdi
Calls a function located at a7 (<<) with %rdi and %xxm0 as the 1
st
and 2
nd
arguments
*Essentially this program takes in a user defined radius and outputs the volume of a cylinder whose height is equal to the radius (therefore pi * r^2h == pi * r^3)
a7:
mov $0x0,%eax
ac:
mov -0x8(%rbp),%rcx
b0:
xor %fs:0x28,%rcx
b7:
00 00
b9:
je c0 <main+0xc0>
bb:
callq c0 <main+0xc0>
c0:
leaveq
c1:
retq
return 0;
}
Moves 0 to eax in preparation for int main() to return 0
Deallocates the stack frame with leave instruction and
returns from the stack
File Three
Step 2:
Explain the functionality of the blocks of assembly code.
Blocks of Assembly Code
Explanation of Functionality
0:
push %rbp
1:
mov %rsp,%rbp
4:
sub $0x20,%rsp
8:
mov %fs:0x28,%rax
f:
00 00
11:
mov %rax,-0x8(%rbp)
15:
xor %eax,%eax
17:
movl $0x1,-0xc(%rbp)
1e:
lea 0x0(%rip),%rsi # 25 <main+0x25>
25:
lea 0x0(%rip),%rdi # 2c <main+0x2c>
2c:
callq 31 <main+0x31>
31:
mov %rax,%rdx
34:
mov 0x0(%rip),%rax # 3b <main+0x3b>
3b:
mov %rax,%rsi
3e:
mov %rdx,%rdi
41:
callq 46 <main+0x46>
46:
lea -0x18(%rbp),%rax
4a:
mov %rax,%rsi
4d:
lea 0x0(%rip),%rdi # 54 <main+0x54>
54:
callq 59 <main+0x59>
The first three instructions allocate 48 bytes to the stack
Moves data at %fs:0x28 to %rax
Moves 64 bit value at %rax to -0x8(%rbp)
Xors %eax with itself effectively setting those bits to zero
Moves a value of 1 (32 bit / 4 bytes) to -0xc(%rbp)
Loads the effective address of the instruction pointer to %rsi
Loads the effective address of the instruction pointer to %rdi
Calls a function located at 31 with %rdi and %rsi as the 1
st
and 2
nd
arguments
Moves %rax (the result of the previous function call) to %rdx
Moves the value of the instruction pointer to %rax
Moves the value of %rax to %rsi
Moves the value of %rdx (the result of the previous function call) to %rdi
Calls a function located at 46 with %rdi and %rsi as the 1
st
and 2
nd
arguments
Loads the effective address of -0x18(%rbp) to %rax
Moves %rax tp %rsi
Loads the effective address of the instruction pointer to %rdi
Calls a function located at 59 with %rdi and %rsi as the 1
st
and 2
nd
arguments
59:
mov -0x18(%rbp),%eax
5c:
sub $0x1,%eax
Moves the value at -0x18(%rbp) to the 32 bit eax
Subtracts 1 from the value at %eax
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
Explanation of Functionality
5f:
mov %eax,-0xc(%rbp)
62:
movl $0x1,-0x10(%rbp)
69:
mov -0x18(%rbp),%eax
6c:
cmp %eax,-0x10(%rbp)
6f:
jg e3 <main+0xe3>
71:
movl $0x1,-0x14(%rbp)
78:
mov -0x14(%rbp),%eax
7b:
cmp -0xc(%rbp),%eax
7e:
jg 99 <main+0x99>
80:
lea 0x0(%rip),%rsi # 87 <main+0x87>
87:
lea 0x0(%rip),%rdi # 8e <main+0x8e>
8e:
callq 93 <main+0x93>
93:
addl $0x1,-0x14(%rbp)
97:
jmp 78 <main+0x78>
99:
subl $0x1,-0xc(%rbp)
Moves the value at %eax to -0xc(%rbp)
Moves a 32 bit value of 1 to to -0x10(%rbp)
[69] (START OF 1ST MAIN LOOP)
Moves the value at -0x18(%rbp) to %eax
Compare the value at %eax to -0x10(%rbp)
If the value at -0x10(%rbp) is more than %eax then it jumps to e3
otherwise the following instructions will run
Moves a 32 bit value of 1 to -0x14(%rbp)
[78]
Moves the value at -0x14(%rbp) to %eax
Compares the value at -0xc(%rbp) to %eax
If the value at %eax is more than -0xc(%rbp)
then jumps to 99
otherwise the indented instructions will run
Load the address of the instruction pointer to %rsi
Loads the address of the instruction pointer to %rdi
Calls a function located at 93 with %rdi %rsi as the 1
st
and 2
nd
arguments
Adds 1 to the value at -0x14(%rbp)
Jumps to 78
Subtracts 1 from the value at -0xc(%rbp)
9d:
movl $0x1,-0x14(%rbp)
a4:
mov -0x10(%rbp),%eax
a7:
add %eax,%eax
a9:
sub $0x1,%eax
ac:
cmp %eax,-0x14(%rbp)
af:
jg ca <main+0xca>
b1:
lea 0x0(%rip),%rsi # b8 <main+0xb8>
b8:
lea 0x0(%rip),%rdi # bf <main+0xbf>
bf:
callq c4 <main+0xc4>
c4:
addl $0x1,-0x14(%rbp)
Moves a 32 bit value of 1 to -0x14(%rbp)
Moves the value at -0x10(%rbp) to %eax
Adds the value at %eax to itself
Subtracts 1 from the value at %eax
Compares the value at %eax to -0x14(%rbp)
If the value at -0x14(%rbp) is greater than %eax then jumps to ca
otherwise the indented instructions will run
Loads the address of the instruction
Blocks of Assembly Code
Explanation of Functionality
c8:
jmp a4 <main+0xa4>
ca:
lea 0x0(%rip),%rsi # d1 <main+0xd1>
d1:
lea 0x0(%rip),%rdi # d8 <main+0xd8>
d8:
callq dd <main+0xdd>
dd:
addl $0x1,-0x10(%rbp)
e1:
jmp 69 <main+0x69>
pointer to %rsi
Loads the address of the instruction pointer to %rdi
Calls a function located at c4 with %rdi %rsi as the 1
st
and 2
nd
arguments
Adds 1 to the value at -0x14(%rbp)
Jumps to 69
(
END OF 1ST MAIN LOOP)
e3:
movl $0x1,-0xc(%rbp)
ea:
movl $0x1,-0x10(%rbp)
f1:
mov -0x18(%rbp),%eax
f4:
sub $0x1,%eax
f7:
cmp %eax,-0x10(%rbp)
fa:
jg 171 <main+0x171>
fc:
movl $0x1,-0x14(%rbp)
103:
mov -0x14(%rbp),%eax
106:
cmp -0xc(%rbp),%eax
109:
jg 124 <main+0x124>
10b:
lea 0x0(%rip),%rsi # 112 <main+0x112>
112:
lea 0x0(%rip),%rdi # 119 <main+0x119>
119:
callq 11e <main+0x11e>
11e:
addl $0x1,-0x14(%rbp)
122:
jmp 103 <main+0x103>
Moves a value of 1 to -0xc(%rbp)
Moves a value of 1 to -0x10(%rbp)
[f1] (START OF 2ND MAIN LOOP)
Moves the value at -0x18(%rbp) to %eax
Subtracts the value at %eax by 1
Compares the value at %eax to -0x10(%rbp)
If the value at -0x10(%rbp) is greater than the value at %eax jump to 171
otherwise run the following instructions
[103]
Moves a 32 bit value of 1 to -0x14(%rbp)
Moves the value at -0x14(%rbp) to %eax
Compares the value at -0xc(%rbp) to %eax
If the value at -0xc(%rbp) is greater than the value at %eax jump to 124
otherwise the indented instructions will run
Loads the address of the instruction pointer to %rsi
Loads the address of the instruction pointer to %rdi
Calls a function located at 11e with %rdi %rsi as the 1st and 2nd arguments
Add 1 to the value at -0x14(%rbp)
Jump to 103
124:
addl $0x1,-0xc(%rbp)
128:
movl $0x1,-0x14(%rbp)
12f:
mov -0x18(%rbp),%eax
132:
sub -0x10(%rbp),%eax
135:
add %eax,%eax
137:
sub $0x1,%eax
13a:
cmp %eax,-0x14(%rbp)
Adds the value at -0xc(%rbp) by 1
Moves a value of 1 to -0x14(%rbp)
[12f]
Moves the value at -0x18(%rbp) to %eax
Subtracts the value at %eax by the value at
Blocks of Assembly Code
Explanation of Functionality
13d:
jg 158 <main+0x158>
13f:
lea 0x0(%rip),%rsi # 146 <main+0x146>
146:
lea 0x0(%rip),%rdi # 14d <main+0x14d>
14d:
callq 152 <main+0x152>
152:
addl $0x1,-0x14(%rbp)
156:
jmp 12f <main+0x12f>
158:
lea 0x0(%rip),%rsi # 15f <main+0x15f>
15f:
lea 0x0(%rip),%rdi # 166 <main+0x166>
166:
callq 16b <main+0x16b>
16b:
addl $0x1,-0x10(%rbp)
16f:
jmp f1 <main+0xf1>
171:
mov $0x1,%eax
176:
mov -0x8(%rbp),%rcx
17a:
xor %fs:0x28,%rcx
181:
00 00
183:
je 18a <main+0x18a>
185:
callq 18a <main+0x18a>
18a:
leaveq
18b:
retq
-0x10(%rbp)
Adds the value of %eax to itself
Subtract 1 from the value at %eax
If the value at -0x14(%rbp) is greater than the value at %eax jump to 158
otherwise the indented instructions will run
Loads the effective address of the instruction pointer to %rsi
Loads the effective address of the instruction pointer to %rdi
Calls a function located at 152 with %rdi and %rsi as the 1
st
and 2
nd
arguments
Adds the value at -0x14(%rbp) by 1
Jump to 12f
Loads the effective address of the instruction pointer to %rsi
Loads the effective address of the instruction pointer to %rdi
Calls a function located at 16b with %rdi and %rsi as the 1st and 2nd arguments
Adds 1 to the value at -0x10(%rbp)
Jump to f1
END OF 2ND MAIN LOOP
Calls a function at 18a if the data at %fs:0x28 was
changed
Deallocates the stack frame and returns the function
Step 4: Convert the assembly code to C++ code.
Step 5: Explain how the C++ code performs the same tasks as the blocks of assembly code.
Blocks of Assembly Code
C++ Code
Explanation of Functionality
0:
push %rbp
1:
mov %rsp,%rbp
4:
sub $0x20,%rsp
8:
mov %fs:0x28,%rax
f:
00 00
11:
mov %rax,-0x8(%rbp)
15:
xor %eax,%eax
17:
movl $0x1,-0xc(%rbp)
1e:
lea 0x0(%rip),%rsi # 25 <main+0x25>
25:
lea 0x0(%rip),%rdi # 2c <main+0x2c>
2c:
callq 31 <main+0x31>
#include <iostream>
int main() {
int xc = 1;
int x18;
std::cout << "Enter number of rows" << std::endl;
std::cin >> x18;
Allocates 32 bytes to the stack
Moves a 32 bit value of 1 to 0xc(%rbp) the stack location for int xc.
Loads the effective address of the instruction pointer (location
of some pointer in this case to null terminated string data “Enter number of rows“) to the 64 bit rsi register.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
C++ Code
Explanation of Functionality
31:
mov %rax,%rdx
34:
mov 0x0(%rip),%rax # 3b <main+0x3b>
3b:
mov %rax,%rsi
3e:
mov %rdx,%rdi
41:
callq 46 <main+0x46>
46:
lea -0x18(%rbp),%rax
4a:
mov %rax,%rsi
4d:
lea 0x0(%rip),%rdi # 54 <main+0x54>
54:
callq 59 <main+0x59>
Loads the effective address of the instruction pointer (location
of some pointer in this case to std::cout) to the 64 bit rdi register.
Calls a function located at 31 (<<) with %rdi and %rsi as the 1st and 2nd arguments
Moves the value at %rax (the value of the previous function call) to %rdx
Moves the value of the instruction pointer (location of some pointer in this case to std::endl) to the 64 bit rsi register.
Moves the value at %rdx (the value of the previous function call) to %rdi
Calls a function located at 46 (<<) with %rdi and %rsi as the 1st and 2nd arguments
Loads the effective address of -0x18(%rbp) (location of stack variable int x18)
to %rax
Moves the value at %rax to %rsi
Loads the effective address of the instruction pointer (location
of some pointer in this case to std::cin) to the 64 bit rdi register.
Calls a function located at 46 (>>) with %rdi and %rsi as the 1st and 2nd arguments
59:
mov -0x18(%rbp),
%eax
5c:
sub $0x1,%eax
5f:
mov %eax,-0xc(%rbp)
62:
movl $0x1,-
0x10(%rbp)
xc = x18 - 1;
//loop from 1 - num_rows
for (int x10 = 1; x10 <= x18; x10++) {
// … } xc = 1; x10 = 1
Moves value at x18 to %eax
Subtracts 1 from the value at %eax
Moves the value at eax to -0xc(%rbp) (location of stack variable int xc)
Blocks of Assembly Code
C++ Code
Explanation of Functionality
69:
mov -0x18(%rbp),
%eax
6c:
cmp %eax,-
0x10(%rbp)
6f:
jg e3 <main+0xe3>
71:
movl $0x1,-
0x14(%rbp)
78:
mov -0x14(%rbp),
%eax
7b:
cmp -0xc(%rbp),%eax
7e:
jg 99 <main+0x99>
80:
lea 0x0(%rip),%rsi # 87 <main+0x87>
87:
lea 0x0(%rip),%rdi # 8e <main+0x8e>
8e:
callq 93 <main+0x93>
93:
addl $0x1,-0x14(%rbp)
97:
jmp 78 <main+0x78>
99:
subl $0x1,-0xc(%rbp)
//loop from 1 - xc
for (int x14 = 1; x14 <= xc; x14+
+) {
// x14++; }
std::cout << " ";
}
xc--;
Moves 32 bit value of 1 to -0x10(%rbp) (location of stack variable int x10)
[START FIRST MAIN LOOP]
Moves value of x18 to %eax
In a for loop because at the end of the loop (dd), the x10 variable is incremented by 1 and there is jump back to 69
. The for loop ends when the value of x10 is greater than x18
Moves 32 bit value of 1 to -0x14(%rbp) (location of stack variable int x14)
In a for loop because at the end of the loop (93), the x14 variable is incremented by 1 and there is jump back to 78
. The for loop ends when the value of x14 is greater than xc
Loads the effective address of the instruction pointer (location of some pointer in this case to null terminated string data “ “) to the 64 bit rsi
register.
Loads the effective address of the instruction pointer (location of some pointer in this case to std::cout) to the 64 bit rdi register.
Calls a function located at 93 (<<) with %rdi and
%rsi as the 1st and 2nd arguments
Subtracts 1 from the value of xc
9d:
movl $0x1,-
0x14(%rbp)
for (int x14 = 1; x14 <= x10 + x10 -1; x14++) {
Sets x14 to a value of 1
Blocks of Assembly Code
C++ Code
Explanation of Functionality
a4:
mov -0x10(%rbp),
%eax
a7:
add %eax,%eax
a9:
sub $0x1,%eax
ac:
cmp %eax,-
0x14(%rbp)
af:
jg ca <main+0xca>
b1:
lea 0x0(%rip),%rsi # b8 <main+0xb8>
b8:
lea 0x0(%rip),%rdi # bf <main+0xbf>
bf:
callq c4 <main+0xc4>
c4:
addl $0x1,-0x14(%rbp)
c8:
jmp a4 <main+0xa4>
ca:
lea 0x0(%rip),%rsi # d1 <main+0xd1>
d1:
lea 0x0(%rip),%rdi # d8 <main+0xd8>
d8:
callq dd <main+0xdd>
dd:
addl $0x1,-0x10(%rbp)
e1:
jmp 69 <main+0x69>
std::cout << "*";
} // x14++
std::cout << std::endl;
} // x10++
Moves value of x10 to %eax
Adds the value at %eax to itself
Subtracts 1 from %eax
In a for loop because at the end of the loop (c4), the x14 variable is incremented by 1 and there is jump back to a4
. The for loop ends when the value of x14 is greater than the value at %eax (x10*2 – 1)
Loads the effective address of the instruction pointer (location of some pointer in this case to null terminated string data “*“) to the 64 bit rsi register.
Loads the effective address of the instruction pointer (location of some pointer in this case to std::cout) to the 64 bit rdi register.
Calls a function located at c4 (<<) with %rdi and %rsi as the 1st and 2nd arguments
Loads the effective address of the instruction pointer (location
of some pointer in this case to std::endl) to the 64 bit rsi register.
Loads the effective address of the instruction pointer (location
of some pointer in this case to std::cout) to the 64 bit rdi register.
Calls a function located at dd (<<) with %rdi and %rsi as the 1st and 2nd arguments
Add 1 to x10
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
C++ Code
Explanation of Functionality
[END FIRST MAIN LOOP]
e3:
movl $0x1,-0xc(%rbp)
ea:
movl $0x1,-
0x10(%rbp)
f1:
mov -0x18(%rbp),
%eax
f4:
sub $0x1,%eax
f7:
cmp %eax,-
0x10(%rbp)
fa:
jg 171 <main+0x171>
fc:
movl $0x1,-
0x14(%rbp)
103:
mov -0x14(%rbp),
%eax
106:
cmp -0xc(%rbp),%eax
109:
jg 124 <main+0x124>
10b:
lea 0x0(%rip),%rsi # 112 <main+0x112>
112:
lea 0x0(%rip),%rdi # 119 <main+0x119>
119:
callq 11e <main+0x11e>
11e:
addl $0x1,-0x14(%rbp)
122:
jmp 103 <main+0x103>
// second half of diamond
xc = 1;
x10 = 1;
for (x10 <= x18 – 1; x10++) {
x14 = 1;
for (xc <= x14; x14++
std::cout << “*”;
}
Moves a value of 1 to xc
Moves a value of 1 to x10
[START 2ND MAIN LOOP]
Moves value of x18 to %eax
Subtracts 1 from value at %eax
In a for loop because at the end of the loop (16b), the x10 variable is incremented by 1 and there is jump back to f1
. The for loop ends when the value of x10 is greater than the value at %eax (x18 – 1)
Move a value of 1 to x14
In a for loop because at the end of the loop (16b), the x10 variable is incremented by 1 and there is jump back to 103
. The for loop ends when the value of x10 is greater than the value at %eax (x18 – 1)
Loads the effective address of the instruction pointer (location of some pointer in this case to null terminated string data “*“) to the 64 bit rsi register.
Loads the effective address of the instruction pointer (location of some pointer in this case to std::cout) to the 64 bit rdi register.
Calls a function located at 11e (<<) with %rdi and %rsi as the 1st and 2nd arguments
124:
addl $0x1,-0xc(%rbp)
128:
movl $0x1,-
xc++;
for (int x14 = 1; x14 <= ((x18 - Increments the value at xc by 1
Sets the value of x14 to 1
Blocks of Assembly Code
C++ Code
Explanation of Functionality
0x14(%rbp)
12f:
mov -0x18(%rbp),
%eax
132:
sub -0x10(%rbp),%eax
135:
add %eax,%eax
137:
sub $0x1,%eax
13a:
cmp %eax,-
0x14(%rbp)
13d:
jg 158 <main+0x158>
13f:
lea 0x0(%rip),%rsi # 146 <main+0x146>
146:
lea 0x0(%rip),%rdi # 14d <main+0x14d>
14d:
callq 152 <main+0x152>
152:
addl $0x1,-0x14(%rbp)
156:
jmp 12f <main+0x12f>
158:
lea 0x0(%rip),%rsi # 15f <main+0x15f>
15f:
lea 0x0(%rip),%rdi # 166 <main+0x166>
166:
callq 16b <main+0x16b>
16b:
addl $0x1,-0x10(%rbp)
16f:
jmp f1 <main+0xf1>
171:
mov $0x1,%eax
176:
mov -0x8(%rbp),%rcx
17a:
xor %fs:0x28,%rcx
181:
00 00
183:
je 18a <main+0x18a>
185:
callq 18a <main+0x18a>
18a:
leaveq
18b:
retq
x10) * 2 - 1); x14++) {
std::cout << "*";
}
std::cout << std::endl;
}
return 0;
}
Moves the value of x18 to eax
Subtracts the value at eax by the value of x10
Adds the value of eax to itself (same as multiplying by 2
Subtracts 1 from eax
In a for loop because at the end of the loop (152), the x14 variable is incremented by 1 and there is jump back to 12f
. The for loop ends when the value at %eax (x18 – x10) * 2 - 1) is greater than the value at x14
Loads the effective address of the instruction pointer (location of some pointer in this case to null terminated string data “*“) to the 64 bit rsi register.
Loads the effective address of the instruction pointer (location of some pointer in this case to std::cout) to the 64 bit rdi register.
Calls a function located at 152 (<<) with %rdi and %rsi as the 1st and 2nd arguments
Loads the effective address of the instruction pointer (location
of some pointer in this case to std::endl) to the 64 bit rsi register.
Loads the effective address of the instruction pointer (location
of some pointer in this case to std::cout) to the 64 bit rdi register.
Calls a function located at 16b
Blocks of Assembly Code
C++ Code
Explanation of Functionality
(<<) with %rdi and %rsi as the 1st and 2nd arguments
[END 2ND MAIN LOOP]
Moves a value of 0 to %eax
Returns the function
File Four
Step 2:
Explain the functionality of the blocks of assembly code.
Blocks of Assembly Code
Explanation of Functionality
;l
The first three instructions allocate 48 bytes to the stack
Moves data at %fs:0x28 to %rax
Moves 64 bit value at %rax to -0x8(%rbp)
Xors %eax with itself effectively setting those bits to zero
Moves a 64 bit value of zero to -20(%rbp)
Moves a 64 bit value of 1 to -0x18(%rbp)
Loads the effective address of the instruction pointer to %rsi
Loads the effective address of the instruction pointer to %rdi
Calls a function located at 3a with %rdi and %rsi as the 1st and 2nd arguments
Moves %rax (the value of the previous function call) to %rdx
Moves the value of the instruction pointer to %rax
Moves the value at %rax to %rsi
Moves the value at %rdx to %rdi
Calls a function located at 4f with %rdi and %rsi as the 1st and 2nd arguments
Loads the effective address of -0x28(%rbp)
to %rax
Moves the value at %rax to %rsi
Loads the effective address of the instruction pointer to %rdi
Calls a function located at 62 with %rdi and %rsi
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
Explanation of Functionality
as the 1st and 2nd arguments
[START OF MAIN LOOP]
Moves the value at -0x28(%rbp) to %eax
Tests the value at %rax to itself. This performs a bitiwise AND operation and raises the zero flag only if %rax is zero
If the zero flag is raised jump to f2
otherwise run the remaining instructions
Move value at -0x28(%rbp) to 64 bit rcx register
Moves a 64 bit value of 0x6666666666666667 to
%rdx
Moves the value at %rcx to %rax
Multiplies the value at %rdx by the value at %rax (-0x28(%rbp))
Shifts all bits at %rdx to the right by 2 effectively dividing it by 2^2 or 4
Subtracts the value at %rdx by the value at %rax
Moves the value of %rdx to %rax
Moves the value at %rax to -0x10(%rbp)
Moves the value at -0x10(%rbp) to %rdx
Moves the value at %rdx to %rax
Shifts all bits at %rax to the left by 2 effectively multiplying it by 2^2 or 4
Adds the value at %rdx to the value at %rax
Adds the value at %rax to itself
Subtracts the value at %rcx by the value at %rax
Moves the value at %rcx to %rax
Moves the value at %rax to -0x10(%rbp)
Moves -0x10(%bp) to %rax
Multiplies the value at %rax by the value at
-0x18(%rbp)
Adds the value at -0x20(%rbp) by %rax
Shifts the value at -0x18(%rbp) to the left by 1
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
Explanation of Functionality
effectively multiplying it by 2
Moves the value at -0x28(%rbp) to %rcx
Moves the 64 bit value of 0x6666666666666667 to %rdx
Moves the value at %rcx to %rax
Multiplu the value at %rdx by the value at %rax
Shift all bits at %rdx 2 places to the right, effectively dividing it by 2^2 or 4
Moves the value at %rcx to %rax
Shifts the bits at %rax 63 places to the right, essentially setting the value of %rax to the most significant bit (in signed values this bit represents
the signedness)
Subtracts the value at %rdx by the value at %rax
Moves the value at %rdx to %rax
Moves the value at %rax to -0x20(%rbp)
Jumps to 62
[END OF MAIN LOOP]
Loads the address of the instruction pointer to %rsi
Loads the address of the instruction pointer to %rdx
Calls a function located at 105 with %rdi and %rsi as the 1
st
and 2
nd
arguments
Moves %rax (the value of the previous function call) to %rdx
Moves the value at -0x20(%rbp) to %rax
Moves the value at %rax to %rsi
Calls a function located at 117 with %rdi and %rsi as the 1
st
and 2
nd
arguments
Moves %rax (the value of the previous function call) to %rdx
Moves the value of the instruction pointer to %rax
Moves the value at %rax to %rsi
Moves the value at %rdx to %rdi
Calls a function located at 12c with %rdi and %rsi
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
Explanation of Functionality
as the 1
st
and 2
nd
arguments
Moves a value of 0 to the eax register
Checks that the value at -0x8(%rbp) is equal to the value at %fs:0x28. If it was modified then a function at 145 will be called otherwise the function deallocates the stack frame and gets returned.
Step 4: Convert the assembly code to C++ code.
Step 5: Explain how the C++ code performs the same tasks as the blocks of assembly code.
Blocks of Assembly Code
C++ Code
Explanation of Functionality
0:
push %rbp
1:
mov %rsp,%rbp
4:
sub $0x30,%rsp
8:
mov %fs:0x28,%rax
f:
00 00
11:
mov %rax,-0x8(%rbp)
15:
xor %eax,%eax
17:
movq $0x0,-
0x20(%rbp)
1e:
00
1f:
movq $0x1,-
0x18(%rbp)
26:
00
27:
lea 0x0(%rip),%rsi # 2e <main+0x2e>
2e:
lea 0x0(%rip),%rdi # 35 <main+0x35>
35:
callq 3a <main+0x3a>
3a:
mov %rax,%rdx
3d:
mov 0x0(%rip),%rax # 44 <main+0x44>
44:
mov %rax,%rsi
47:
mov %rdx,%rdi
4a:
callq 4f <main+0x4f>
4f:
lea -0x28(%rbp),%rax
53:
mov %rax,%rsi
56:
lea 0x0(%rip),%rdi # 5d <main+0x5d>
5d:
callq 62 <main+0x62>
#include <iostream>
int main()
{
long x28;
long x20 = 0;
long x18 = 1;
std::cout << "Enter the binary number:" << std::endl;
std::cin >> x28;
Allocates 42 bytes of data to the
stack frame (start of int main())
Moves a 64 bit value of 0 to -0x20(%rbp) which is the location of the stack variable long x20.
In C++ most compilers have long as a variable type that can hold 64 bits.
Moves a 64 bit value of 1 to -0x18(%rbp) (long x18)
Loads the effective address of the instruction pointer (location
of some pointer in this case to null terminated string data “Enter the binary number:“) to the 64 bit rsi register.
Loads the effective address of the instruction pointer (location
of some pointer in this case to std::cout) to the 64 bit rdi register.
Calls a function located at 3a (<<) with %rdi and %rsi as the 1
st
and 2
nd
arguments
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
C++ Code
Explanation of Functionality
Moves the result of the function
call to %rdx
Moves the value of the the instruction pointer (location of some pointer in this case to std::endl) to the 64 bit rax register.
Moves the value of %rax to %rsi
Moves the value at %rdx (the value of the previous function call) to %rdi
Calls a function located at 4f with %rdi and %rsi as the 1
st
and
2
nd
arguments.
Loads the effective address of -0x28 (the address of the stack variable long x28) to %rax
Moves the value at %rax to %rsi
Loads the effective address of the instruction pointer (location
of some pointer in this case to std::cin) to the 64 bit rdi register.
Calls a function located at 62 (>>) with %rdi and %rsi as the 1
st
and 2
nd
arguments
62:
mov -0x28(%rbp),
%rax
66:
test %rax,%rax
69:
je f2 <main+0xf2>
6f:
mov -0x28(%rbp),%rcx
73:
movabs $0x6666666666666667,%rdx
7a:
66 66 66
7d:
mov %rcx,%rax
80:
imul %rdx
83:
sar $0x2,%rdx
87:
mov %rcx,%rax
8a:
sar $0x3f,%rax
8e:
sub %rax,%rdx
while (x28 != 0) {
x10 = ((x28 * 0x6666666666666667) >> 0x2) -
(x28 >> 0x3f);
x10 = x28 - (((x10 << 0x2) + x10) * 2);
**Starts while loop at 62 because a later instruction jumps back to 62 and there is a conditional jump at 69 that exits the loop.
[START OF MAIN LOOP]
Moves the value at x28 to %rax
Tests the value at %rax to itself. This performs a bitiwise AND operation and raises the zero flag only if %rax is zero
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
C++ Code
Explanation of Functionality
91:
mov %rdx,%rax
94:
mov %rax,-
0x10(%rbp)
98:
mov -0x10(%rbp),
%rdx
9c:
mov %rdx,%rax
9f:
shl $0x2,%rax
a3:
add %rdx,%rax
a6:
add %rax,%rax
a9:
sub %rax,%rcx
ac:
mov %rcx,%rax
af:
mov %rax,-
0x10(%rbp)
If the zero flag is raised jump to f2
otherwise run the remaining instructions
Moves the value at x28 to %rcx
Moves the 64 bit value 0x6666666666666667 to %rdx
Moves the data at %rcx (x28) to %rax
Multiplies the value at %rdx by the value at %rax (x28)
Shifts the value at %rdx to the right by 2
Moves the value at %rcx (x28) into the rax register
Shift the bits in the %rax by 0x3f
Subtract the value at %rdx by %rax
Moves the value at %rdx to -0x10(%rbp) (long x10)
Moves the value at x10 tp %rdx
Moves the value at %rdx tp %rax
Shifts the bits at %rdx (x10) to the left by 2
Adds the value at %rdx to the value at %rax
Adds the value at %rax to itself effectively multiplying it by 2
Subtracts the value at %rcx (x28) by the value at %rax
Moves the value at %rcx to %rax
Moves the value at %rax to
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
C++ Code
Explanation of Functionality
-0x10(%rbp)
b3:
mov -0x10(%rbp),
%rax
b7:
imul -0x18(%rbp),%rax
bc:
add %rax,-0x20(%rbp)
c0:
shlq -0x18(%rbp)
c4:
mov -0x28(%rbp),%rcx
c8:
movabs $0x6666666666666667,%rdx
cf:
66 66 66
d2:
mov %rcx,%rax
d5:
imul %rdx
d8:
sar $0x2,%rdx
dc:
mov %rcx,%rax
df:
sar $0x3f,%rax
e3:
sub %rax,%rdx
e6:
mov %rdx,%rax
e9:
mov %rax,-
0x28(%rbp)
ed:
jmpq 62 <main+0x62>
x20 += (x10 * x18);
x18 = (x18 << 1);
x28 = ((0x6666666666666667 * x28) >> 0x2) - (x28 >> 0x3f);
}
Moves the value of x10 to %rax
Multiplies the value at %rax (x10) by x18
Add the value at %rax to -0x20(%rbp)
Shifts the bits at -0x18(%rbp) (x18) to the left by 1
Moves the value of x28 to %rcx
Move the 64 bit value of 0x6666666666666667 to %rdx
Move the value at %rcx (x28) to %rax
Multiplies the value at %rdx by the value at %rax (x28)
Shifts the value at %rdx to the right by 2
Moves the value at %rcx (x28) to %rax
Shifts the bits of the value at %rax (x28) 63 places to the right
Subtracts the value at %rdx by the value at %rax
Moves the value at %rdx to %rax
Moves the value at %rax to
-0x28(%rbp)
Jumps to 62
[END OF MAIN LOOP]
f2:
lea 0x0(%rip),%rsi # f9 <main+0xf9>
f9:
lea 0x0(%rip),%rdi # 100 <main+0x100>
100:
callq 105 <main+0x105>
105:
mov %rax,%rdx
108:
mov -0x20(%rbp),
%rax
10c:
mov %rax,%rsi
10f:
mov %rdx,%rdi
std::cout << "Equivalent hexadecimal value:" << x20 << std::endl;
Loads the effective address of the instruction pointer (location
of some pointer in this case to null terminated string data “Equivalent hexadecimal value:“) to the 64 bit rsi register.
Loads the effective address of the instruction pointer (location
of some pointer in this case to
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Blocks of Assembly Code
C++ Code
Explanation of Functionality
112:
callq 117 <main+0x117>
117:
mov %rax,%rdx
11a:
mov 0x0(%rip),%rax # 121 <main+0x121>
121:
mov %rax,%rsi
124:
mov %rdx,%rdi
127:
callq 12c <main+0x12c>
std::cin) to the 64 bit rdi register.
Calls a function located at 105 (<<) with %rdi and %rsi as the 1
st
and 2
nd
arguments
Moves %rax (the value of the previous function call) to %rdx
Moves the value of x20 to %rax
Moves the value at %rax to %rsi
Moves the value at %rdx to %rdi
Calls a function located at 117 (<<) with %rdi and %rsi as the 1
st
and 2
nd
arguments
Moves %rax (the value of the previous function call) to %rdx
Moves the value of the instruction pointer (location of some pointer in this case to std::endl) to the 64 bit rax register.
Moves the value at %rax to %rsi
Moves the value at %rdx (the value of the previous function call) to %rdi
Calls a function located at 12c (<<) with %rdi and %rsi as the 1
st
and 2
nd
arguments
12c:
mov $0x0,%eax 131:
mov -0x8(%rbp),%rsi
135:
xor %fs:0x28,%rsi
13c:
00 00
13e:
je 145 <main+0x145>
140:
callq 145 <main+0x145>
145:
leaveq
146:
retq
return 0;
}
Moves a value of 0 to the eax register in preparation of int main() returning a zero
Deallocates the stack frame ( } ) and returns the function
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Recommended textbooks for you
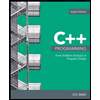
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
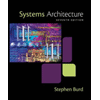
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
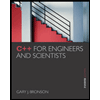
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
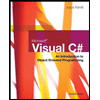
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
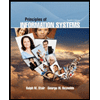
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
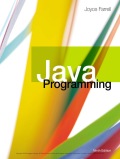
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Principles of Information Systems (MindTap Course...Computer ScienceISBN:9781285867168Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
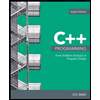
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
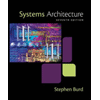
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
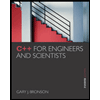
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
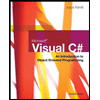
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
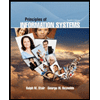
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
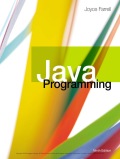
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT