6470506_2027090555_2051684520957 (1)
pdf
keyboard_arrow_up
School
Multimedia University of Kenya *
*We aren’t endorsed by this school
Course
NETWORKS
Subject
Computer Science
Date
Nov 24, 2024
Type
Pages
14
Uploaded by MasterGazelle3536
Cybersecurity Education Labs
Off-Path TCP Exploits Lab
Table of Contents
1.
Overview
...........................................................................
3
2.
Virtual Machine Setup
..........................................................
4
3.
Lab Task Set 1: Clock Synchronization
....................................
6
4.
Lab Task Set 2: Four-Tuple Inference
......................................
9
5.
Lab Task Set 3: Sequence Number Inference and Nash Equilibrium10
6.
Lab Task Set 4: Acknowledgement Number Inference
................
12
7.
Submission
........................................................................
13
References
.............................................................................
14
Beacon Labs: Network Security
2 of 14
Off-Path TCP Exploits Lab
1
Overview
This attack is executed on Linux Kernel 3.13. In Linux Kernel 3.6 RFC 5961
was faithfully implemented to stop blind in-window attacks, but it also cre-
ated a new vulnerability. RFC 5961 proposed a window outside of the cor-
rect ACK-window where the server would respond with a challenge-ACK
to packets that did not have the correct sequence or ACK number. Figure 1
demonstrates this below. To prevent excess resources from being used on
these challenge-ACKs, a limit of 100 per second was implemented, known
as the Global Rate Limit. This limit is where the vulnerability lies.
Figure 1: ACK Window Illustration
The Global Rate Limit can be exploited to show if a TCP connection is
present, and then subsequently be used to infer the four-tuple of the client
and server, infer the next acceptable sequence number, then finally infer
the acknowledgment number. After this is completed it is trivial to inject
spoofed packets.
Readings and Videos:
Video explaining how the attack works:
https://www.usenix.org/conference/usenixsecurity16/technical-sessions/presentation/
cao
Paper that the attack is based upon:
https://www.cs.ucr.edu/~zhiyunq/pub/sec16_TCP_pure_offpath.pdf
Beacon Labs: Network Security
3 of 14
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Off-Path TCP Exploits Lab
The objective of this lab is to have students understand how this attack is
carried out and write some of the code necessary to execute it.
2
Virtual Machine Setup
In this lab we will have three virtual machines: the client, server, and at-
tacker. There are two virtual machines to download as the client and at-
tacker use the same image. Through a python script to create a simple
messaging service between the client and server, we create the TCP con-
nection that will be hijacked.
The server is running a version of Ubuntu 14.04 that has not been updated.
The security updates for this operating system patch the vulnerability that
we are exploiting, so we must be sure not to update it.
The client and the attacker are running Ubuntu 16.04. The attacker also
has the libtins libraries installed.
They are the libraries that we use to
spoof and send packets, so they are necessary to have on the virtual ma-
chine.
By interacting with the server as seen in figure 2, we can complete all steps
of the attack. After this is completed we can then inject packets imitating
the server to the client, as seen in figure 3.
Figure 2: Exploiting Server
Figure 3: Imitating the Server
Make sure all virtual machines only have a Host-Only Adapter in their net-
work adapters.
2.1 Connecting Client and Server
Beacon Labs: Network Security
4 of 14
Off-Path TCP Exploits Lab
On the desktop of the server and client there is a file labeled tcp_server.py
and tcp_client.py respectively. The code requires the window to be larger
than the standard size, so put the terminal in full screen before executing
commands. Through the terminal navigate to the desktop on the server
first and run the code via the command:
$ sudo python3 tcp_server.py <server_ip> <server_port>
// Find your server ip and use it as an argument
// Choose a port to host the connection on. Make sure no other connec-
tion are using that port
Once this is complete your server terminal should look like figure 4.
Figure 4: Server Waiting for Connection
Once that is complete, we can connect the client to the server. Navigate to
the desktop again and run tcp_client.py using the same arguments:
$ python3 tcp_client.py <server_ip> <server_port>
Your client terminal should look like figure 5, and your server terminal
should look like figure 6.
2.2 Setting up the Attacker
The attacker code is saved in the folder attacker_cpp in documents. When
you alter the code and want to compile it again, remove the file named ex-
ploit, then run the command make in the terminal whilst in the folder.
The attack can then be run from the terminal by typing:
Beacon Labs: Network Security
5 of 14
Off-Path TCP Exploits Lab
Figure 5: Client Terminal after Connec-
tion
Figure 6: Server Terminal after Connec-
tion
$ sudo ./exploit <client_ip> <server_ip> <server_port>
The server port will be the same as the one used to set up the tcp connec-
tion. The attack will not work yet though as it is missing code that will be
added by you. After attempting the attack on the tcp connection, further
attacks on the same connection may be slow or unsuccessful.
Restart-
ing the tcp connection of the server and client on a new port will mitigate
this.
3
Lab Task Set 1: Clock Synchronization
To make sure all of our packets arrive within the same time interval, we
must first synchronize our clock with the server. This is done by first initiat-
ing a legitimate TCP connection. In synchronize_clock.cpp (Figure 7) you
must choose what flags should be sent to create this connection.
Write what flag should be set to first initialize the TCP connection on line
25, then disable this flag and set another one that will be used after receiv-
ing the response from the server on lines 47 and 48.
Beacon Labs: Network Security
6 of 14
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Off-Path TCP Exploits Lab
Figure 7: synchronize_clock.cpp Step 1
After altering the code, compile it by navigating to the attacker_cpp folder,
removing the exploit.exe file, and then entering the make command.
Once we have our legitimate connection established we must now send
200 packets equally spaced in the span of a second to see how many
challenges this prompts. Since only 100 challenges can be sent within a
second, we know that our clock is synchronized when we only receive 100
challenges.
There is a chance that the clock will already be synchronized, but it is far
more likely that we will end up receiving more than 100 challenges. We
try this twice and adjust the time between the first and second round. The
third round uses the calculated time delay necessary to receive only 100
challenges. Your task is to write code to equally space out the 200 packets
in the span of a second so that each packet has an equal amount of time
between the one before and the one after and use the correct time delay
for round one, two, and three.
The way the code is currently, all packets will be sent as fast as the com-
puter can. Your task is to create a delay between each packet being sent
Beacon Labs: Network Security
7 of 14
Off-Path TCP Exploits Lab
out so that all 200 are equally spaced withing the span of a second, having
the same amount of time between the packet before it and the one after.
Make sure to add the variable "start" to your time delay. Write your code
on line 75 for round one as seen in figure 8.
Figure 8: synchronize_clock.cpp Step 2
In the second round we want to add an additional three seconds to the
time delay to make sure all packets from the last round were responded to
and the global rate limit has been reset. We also want to add another 5
milliseconds to the time delay so that a different number of challenges are
received and we can calculate what the final adjustment of time should be.
Don’t forget to add the start to the time delay again. Write your code on
line 98 for round two as seen in figure 9.
Figure 9: synchronize_clock.cpp Step 3
For the final round we want to send another group of equally spaced pack-
ets with the delay of 6 seconds (3 from the first round, and an additional 3
Beacon Labs: Network Security
8 of 14
Off-Path TCP Exploits Lab
from the second). For this final round though we want to add the variable
of n_result to our time delay to make sure our calculation was correct and
only 100 packets are received in a second. A margin of error of one packet
(101 received) is acceptable. Write your code on line 127 for round three
as seen in figure 10.
Figure 10: synchronize_clock.cpp Step 4
After altering the code, compile it by navigating to the attacker_cpp folder,
removing the exploit.exe file, and then entering the make command. There
is a redundancy in the code to try the time synchronization again in case
there was any packet loss. If the code is written correctly by the student
it should only take one round to complete but there is a possibility it will
need a second or third.
4
Lab Task Set 2: Four-Tuple Inference
Since there is a tcp connection between the client and server, a SYN-
ACK packet sent with that four-tuple will prompt a challenge-ACK. If the
SYN-ACK packet is sent to a port that does not have a tcp connection
taking place, the server will send an RST packet in response. We use this
response to find the correct client port.
Over the course of a second, we send out SYN-ACK packets to the server
with the four-tuples of <srcIP = clientIP, dstIP = serverIP, srcPort =X, dst-
Port = serverPort>. The source port is labeled as X as it is the part that
we need to figure out.
The default linux port range is 32768 to 61000.
By halving this and sending packets from 45000-61000, we can determine
which part of this range holds the correct port number.
Beacon Labs: Network Security
9 of 14
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Off-Path TCP Exploits Lab
Once we have sent out SYN-ACK packets in the first half, we can send 100
rst packets during the same second. If 100 challenge-ACKs are observed,
we know it is in the first half of the search space.
If 99 are observed,
we know one was sent to the client, and thus the port is in the first half.
Write how we use the amount observed to find if the correct client port is
in the range we searched and how we should adjust the right or left port
accordingly. Write your code on line 67 as seen in figure 11.
Figure 11: 4tuple_inference.cpp Step 1
After altering the code, compile it by navigating to the attacker_cpp folder,
removing the exploit.exe file, and then entering the make command. Once
the client port is found we can move on to finding the sequence num-
ber.
5
Lab Task Set 3: Sequence Number Infer-
ence and Nash Equilibrium
Challenge-ACKs are prompted for RST packets that are received if the
sequence number is in the window, but does not exactly match the next
expected sequence number (RCV.NXT). Knowing this, we can break the
sequence number space into blocks of the received window size and send
packets to each block.
To find the correct block, we can either go through the search space by
increasing our block_id, or decreasing it.
Below is a game theory table
(Table 1 representing the advantages and disadvantages of both.
The defender has the option of starting the sequence number on a high
value or a low value. Starting on a high value costs more resources, thus
Beacon Labs: Network Security
10 of 14
Off-Path TCP Exploits Lab
Defender
Start Low
Start High
Attacker
Search Low
(2,
-
2)
(2,
-
2)
Search High
(1,
-
1)
(3,
-
3)
Table 1: Game Theory
it gives the attacker 2 and the defender -2. If the defender starts it on a
low value, then it’s 1 and -1 instead. If the attacker chooses correctly to
search high or low first, then another 1 and -1 is given to the attacker and
defender respectively.
Analyze this game theory table and make a decision of what would be
more advantageous as an attacker:
Searching high or searching low.
Once you have made your decision, write the for loop on line 61 to reflect
it. Give an explanation for your decision in your documentation.
The definitions of the variables are as follows.
SEQ_MAX is the maxi-
mum value a 32 bit int can be as that is the largest sequence number
WINDOW_SIZE is 12,600 as that is the default window size for linux.
chunk_size is 8000 as that is the area we send a packet to each round.
SEQ_MAX/chunk_size/WINDOW_SIZE is the maximum chunk_id we would
search to if counting up from 0.
Three steps must be taken to find the correct sequence number. First by
approximating the range in which the number lies (above), second finding
the correct block, and finally finding the sequence number. In step one,
you must also write code to send packets on the legitimate connection
to exhaust the global rate limit on line 73, and an if statement to check
if the correct block is in the current search space on line 91 as seen in
figure 12.
After altering the code, compile it by navigating to the attacker_cpp folder,
removing the exploit.exe file, and then entering the make command.
In step 2, we narrow down the search space to a single block.
This is
done in a similar method to the 4-tuple inference where we progressively
narrow down our search space by seeing how many packets are received
after sending them to one half. You do not have to write any code for this
Beacon Labs: Network Security
11 of 14
Off-Path TCP Exploits Lab
Figure 12: sequence_inference.cpp Step 1
step.
In step 3 we iterate through sequence numbers until we find the correct
one. Once we have found it we can proceed to inferring the Acknowledge-
ment Number. You do not have to write any code for this step.
6
Lab Task Set 4: Acknowledgement Num-
ber Inference
To find the next expected acknowledgement number of the server, we use
a multi-bin search. The maximum window size for acknowledgement num-
bers is
2
30
. We define this in the code as one_G. By sending one packet
to the 0 sequence number, two to one_G -1, four to two_G -1, and eight to
three_G -1, we can then count the amount of packets received to find the
area in which the correct number lies. For instance, if 94 challenges are
received, then we know one_G and two_G have triggered challenges, thus
the range is in between the two of them. If 96 challenges are observed,
then we know only two_G has triggered challenges, thus the range is in-
side of two_G. Your task is to write how the code will handle missing four
packets on line 89, eight packets on line 100, and 12 packets on line 11 as
seen in figure 13.
Beacon Labs: Network Security
12 of 14
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Off-Path TCP Exploits Lab
Figure 13: ack_inference.cpp
After altering the code, compile it by navigating to the attacker_cpp folder
through the terminal, removing the exploit.exe file, and then entering the
make command.
Once this is completed we can find the left boundary of the acceptable
ACK range, and then create spoofed packets that can be sent to the client.
If all code is working correctly, you should be able to write messages into
the console that will show up as the server in the client’s messaging termi-
nal.
7
Submission
Submit a lab report using the provided
lab report template
. Create a lab
report that consists of text and images from each task in the lab. Describe
the process you went through to solve each task. Be sure to include how
you solved the game theory table. With your submission include the syn-
chronize_clock.cpp, 4tuple_inference.cpp, sequence_inference.cpp, and
Beacon Labs: Network Security
13 of 14
Off-Path TCP Exploits Lab
ack_inference.cpp files.
References
Beacon Labs: Network Security
14 of 14
Related Documents
Recommended textbooks for you
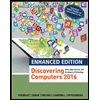
Enhanced Discovering Computers 2017 (Shelly Cashm...
Computer Science
ISBN:9781305657458
Author:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Publisher:Cengage Learning
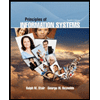
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
Recommended textbooks for you
- Enhanced Discovering Computers 2017 (Shelly Cashm...Computer ScienceISBN:9781305657458Author:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. CampbellPublisher:Cengage LearningPrinciples of Information Systems (MindTap Course...Computer ScienceISBN:9781285867168Author:Ralph Stair, George ReynoldsPublisher:Cengage Learning
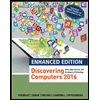
Enhanced Discovering Computers 2017 (Shelly Cashm...
Computer Science
ISBN:9781305657458
Author:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Publisher:Cengage Learning
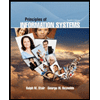
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning